I had to change the design of the ListPicker due to the overlapping on the radio buttons of the Table Type. Have to consider improving this in future. So here the user has to first select a branch, and then select the type of table, which will display the available table numbers.
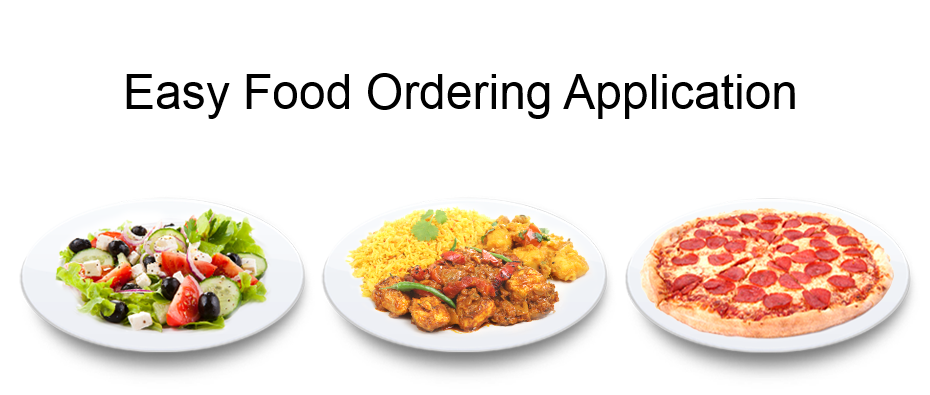
This blog will be used as an online diary to log/post updates on the progress of my work for the Enterprise Development Project (MSc.EAD) module, in partial fulfillment of the MSc. Enterprise Application Development programme offered by Sheffield Hallam University, UK. - S.M.Mohammed Mafaz (PGE/12/0005)
Wednesday, June 19, 2013
Tuesday, June 18, 2013
Day 27: Adjusting ListPicker control
Referred to this article on implementing ListPicker for displaying the branch names and table names on the Select Table page.
Wednesday, June 12, 2013
Day 26: Completion of Menu Selection UI
As initially planned, by the use of Isolated Storage settings was able to store the selected menu where the quantity is more than 0. Need to improve the user friendliness of the quantity textbox, since when tapped on it the keypad with alphabets is appearing. Need to improve this to display the numeric keypad by default.
In overall the functionality of this page is complete.
In overall the functionality of this page is complete.
Thursday, June 6, 2013
Day 25: Menu Display Page ListBox
Got the listbox display the correct menu description and price as we click through the arrow buttons.
And in the MenuDisplayLogic class this is how I retrieve the details of all menus for initializing:
This functionality was implemented with a hint from this article.
I had to override the ToString() method in the Menu class as follows to bind it to the control that displays it.
public class Menu
{
private int _imageIndex;
public int ImageIndex
{
get { return _imageIndex; }
set { _imageIndex = value; }
}
private float _price;
public float Price
{
get { return _price; }
set { _price = value; }
}
private string _menuName;
public string MenuName
{
get { return _menuName; }
set { _menuName = value; }
}
private int _quantity;
private string _code;
public string Code
{
get { return _code; }
set { _code = value; }
}
public int Quantity
{
get { return _quantity; }
set { _quantity = value; }
}
public override string ToString()
{
return " "+MenuName + "\n Rs." + Math.Round(Convert.ToDouble(Price),2).ToString()+"/=";
}
}
public static List<Models.Menu> GetMenuDetails()
{
List<Models.Menu> menuDetails = new List<Models.Menu>();
using (EasyFoodMobDBContext dbcontext = new EasyFoodMobDBContext(EasyFoodMobDBContext.ConnectionString))
{
bool res = dbcontext.CreateIfNotExists();
}
using (EasyFoodMobDBContext dbcontext = new EasyFoodMobDBContext(EasyFoodMobDBContext.ConnectionString))
{
var query = from c in dbcontext.Menu
select new { c.FoodCode,c.FoodDescription,c.FoodPrice };
foreach(var menu in query)
{
Models.Menu newmenu = new Models.Menu();
newmenu.Code = menu.FoodCode;
newmenu.MenuName = menu.FoodDescription;
newmenu.Price = (float)menu.FoodPrice;
menuDetails.Add(newmenu);
}
}
return menuDetails;
}
Then I had to do small modifications to the Carousel control class for being able to initialize the details and display them appropriately as the user browses through the menu. First I set the correct image index to the Menu objects in the initialization. Only through this index I would be able to display the correct details.
private void AddImagesToCarousel()
{
menuList = MenuDisplayLogic.GetMenuDetails();
if (ListImagesCarousel != null && ListImagesCarousel.Count > 0)
{
for (int i = 0; i < ListImagesCarousel.Count; i++)
{
// get the image url
//string url = ListImagesCarousel[i];
Image image = new Image();
//image.Source = new BitmapImage(new Uri(url, UriKind.RelativeOrAbsolute));
image = ListImagesCarousel[i];
// TAG is used as an identifier for images
image.Tag = i.ToString();
//Adding it to Menu
menuList[i].ImageIndex = i;
// event Tapped to find selected image
image.Tap += image_Tap;
// add and set image position
LayoutRoot.Children.Add(image);
SetPosImage(image, i);
_images.Add(image);
}
}
}
Then on the initial state and on other states I would need to get the correct menu, and bind it directly to the control. Thanks to LINQ, it took only a single line of code.
private void SetIndex(int value)
{
_target = value;
_target = Math.Max(0, _target);
_target = Math.Min(_images.Count - 1, _target);
listBox1.ItemsSource = menuList.Where(a => a.ImageIndex == _target);
}
private void MoveIndex(int value)
{
_target += value;
_target = Math.Max(0, _target);
_target = Math.Min(_images.Count - 1, _target);
listBox1.ItemsSource = menuList.Where(a => a.ImageIndex == _target);
}
With this 90% of work for this page is complete. I am only left with enabling the user to enter the quantity of the menu and the saving of the order to the isolated storage.
Tuesday, June 4, 2013
Day 24: Menu Display Logic
Continued to work on the menu display logic. Hope to finish the functionality of this page by tomorrow.
Monday, June 3, 2013
Day 22: Page Navigation
- Referred to this article which explains briefly how to navigate and pass parameters from one page to the another.
Day 23: Tweaks to the Menu Selection UI
- Was working on arranging the image carousel additionally with a textblock & textbox to store the quantity of each menu ordered. This cannot be added in the Main control since the events related to the picture browsing were in the Carousel control, and the quantity ordered information needs to be synchronizing with the picture swiping. The carousel control was not aligning properly when new controls were added to it.
- Read articles on serializing and sending objects over WCF.
Subscribe to:
Posts (Atom)